views
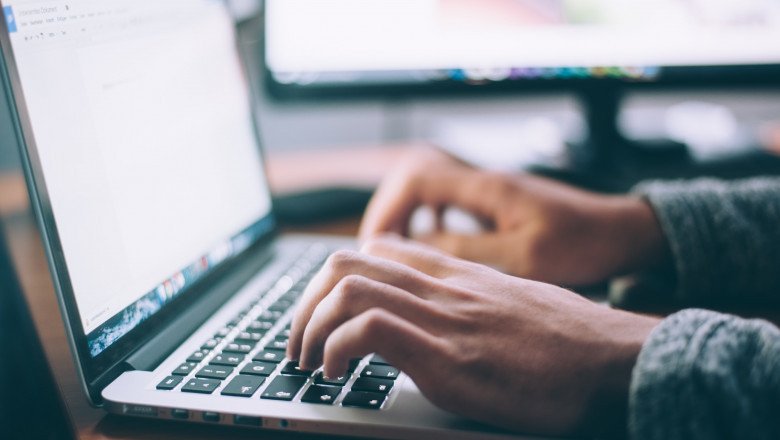
Real estate is a huge market, and a well-designed real estate listing application can be a valuable tool for both buyers and sellers. For buyers, it allows them to search for properties, view information about them, and compare different listings. For sellers, it provides a platform to showcase their properties to a wider audience.
The purpose of this article is to provide a comprehensive guide on how to build a real estate listing application using ReactJS and Zillow API. This article will cover everything from setting up the development environment to deploying the application and will be helpful for both beginners and experienced developers. By the end of the article, you will have a working real estate listing application that you can further customize and enhance to meet your needs.
Understanding the Zillow API
A. Overview of the Zillow API:
Zillow is one of the largest real estate websites in the world and it provides a wealth of information about properties for sale or rent. The Zillow API allows developers to access this data and use it in their own applications. The API provides a wide range of information about properties, including the property's address, details about the property, images, estimated value, and more. This data is updated regularly, so it is always up-to-date and accurate.
B. Benefits of using the Zillow API for Real-Estate Listing Applications:
There are many benefits to using the Zillow API for real estate listing applications, including:
-
Rich and Up-to-date Data: Zillow has a vast amount of data about properties and is constantly updating this information. This means that developers can access accurate and up-to-date information about properties, making it easier to build a comprehensive real estate listing application.
-
User-Friendly API: The Zillow API is easy to use, with a well-documented API reference and a range of code samples to help developers get started. This makes it accessible even to developers who are new to working with APIs.
-
Large Community of Developers: Zillow has a large and active community of developers who use the API, so it is easy to find help and support when needed. This can be especially helpful for developers who are new to building real estate listing applications.
-
Cost-Effective: The Zillow API is free to use, which makes it an attractive option for developers who are building real estate listing applications on a budget.
C. How to access the Zillow API:
Accessing the Zillow API is simple, and developers can get started in just a few steps. Here is how to get started:
-
Register for an API Key: To use the Zillow API, developers must first register for an API key. This can be done on the Zillow website by creating an account and applying for an API key.
-
Review the API Documentation: Once you have an API key, it is important to review the API documentation to understand how the API works and what data it provides.
-
Test the API: Before using the API in your application, it is a good idea to test it to make sure it works as expected. This can be done using a tool like Postman, which allows you to test API requests and see the results.
-
Integrate the API into your Application: Once you have tested the API and are familiar with how it works, you can integrate it into your real estate listing application. This will typically involve writing code to make API requests and process the data returned by the API.
In conclusion, the Zillow API is a powerful tool for building real estate listing applications, offering rich and up-to-date data, a user-friendly API, a large community of developers, and a cost-effective solution. To get started, developers must first register for an API key, review the API documentation, test the API, and then integrate it into their application.
Setting up the ReactJS Environment
A. Introduction to ReactJS:
ReactJS is a popular JavaScript library for building user interfaces. It was developed by Facebook and is widely used in web development. ReactJS allows developers to build reusable UI components, making it easier to create complex user interfaces. This makes it an ideal choice for building real estate listing applications, as it allows developers to create a customized, visually appealing interface for users.
B. Installing and setting up ReactJS:
Getting started with ReactJS is simple, and developers can get up and running in just a few steps. Here is how to set up ReactJS:
-
Install Node.js: ReactJS is built using Node.js, so the first step is to install Node.js on your development machine.
-
Create a ReactJS Project: To create a ReactJS project, you can use the "npx create-react-app" command. This will create a new ReactJS project with a basic file structure and set up all the necessary dependencies.
-
Start the Development Server: Once your ReactJS project is set up, you can start the development server using the "npm start" command. This will launch your ReactJS application in a web browser, allowing you to view it and make changes.
C. Understanding the basics of ReactJS Components:
ReactJS components are the building blocks of a ReactJS application. Components are reusable UI elements that can be combined to create complex user interfaces. Components can receive data and render it to the screen. There are two types of components in ReactJS: stateful components and stateless components.
-
Stateful Components: Stateful components are components that have internal state, meaning that they can store data and manipulate it over time.
-
Stateless Components: Stateless components are components that do not have internal state and simply receive data as props and render it to the screen.
In ReactJS, components can be nested within other components, allowing developers to build complex user interfaces. This makes it possible to create reusable UI elements that can be used throughout an application.
In conclusion, ReactJS is a powerful tool for building user interfaces and is well-suited for building real estate listing applications. To get started with ReactJS, developers must install Node.js, create a ReactJS project, and start the development server. Understanding the basics of ReactJS components is also essential, as components are the building blocks of a ReactJS application.
Building the ReactJS Application
A. Creating the components for the application:
In this section, we will create the components that make up our real estate listing application. A typical real estate application will have several components, such as a header, footer, search bar, list of properties, and a property detail view.
-
Header Component: The header component will display the title of the application and any other relevant information, such as the number of properties.
-
Footer Component: The footer component will display the copyright information and any other relevant information.
-
Search Bar Component: The search bar component will allow users to search for properties based on various criteria, such as location, price range, and property type.
-
List of Properties Component: The list of properties component will display a list of properties that match the criteria entered by the user in the search bar.
-
Property Detail View Component: The property detail view component will display detailed information about a specific property, such as its location, price, and description.
B. Designing the user interface:
The user interface of the real estate listing application is a crucial aspect of the application. The interface must be visually appealing, easy to navigate, and provide a great user experience. To design the user interface, developers should consider the following:
-
Use of color: The use of color is an important aspect of the user interface. Developers should choose colors that are visually appealing and that match the overall design of the application.
-
Typography: Typography is another important aspect of the user interface. Developers should choose fonts that are easy to read and match the overall design of the application.
-
Layout: The layout of the user interface is also important. Developers should choose a layout that is visually appealing and easy to navigate.
C. Implementing the Zillow API to fetch and display data:
The Zillow API allows developers to retrieve real estate data, such as property information, listings, and details. To implement the Zillow API, developers will need to make an API request to the Zillow API, retrieve the data, and display it in the user interface.
-
Making the API Request: The first step in implementing the Zillow API is to make an API request. This can be done using the Fetch API, which is a built-in JavaScript API for making network requests.
-
Retrieving the Data: Once the API request has been made, the next step is to retrieve the data. The data can be retrieved in JSON format, which can be easily parsed and displayed in the user interface.
-
Displaying the Data: The final step in implementing the Zillow API is to display the data in the user interface. This can be done by using the components created in the previous step.
D. Adding features like searching and filtering:
In this section, we will add features to the real estate listing application, such as searching and filtering.
-
Searching: Searching allows users to search for properties based on various criteria, such as location, price range, and property type. To implement searching, developers can use the search bar component created in the previous step.
-
Filtering: Filtering allows users to filter properties based on specific criteria, such as property type and price range. To implement filtering, developers can use dropdown menus, checkboxes, or other similar UI elements.
In conclusion, building the ReactJS application involves creating components, designing the user interface, implementing the Zillow API to fetch and display data, and adding features like searching and filtering.
Deployment and Testing
A. Deploying the application to a web server:
Once the ReactJS application has been developed, the next step is to deploy it to a web server. Deployment is the process of making the application available for users to access. There are several options for deploying a ReactJS application, including:
-
Hosting on a cloud-based platform: This is a popular option for deployment, as it is easy to set up and maintain. Some popular cloud-based platforms for hosting ReactJS applications include Heroku, AWS, and Google Cloud Platform.
-
Self-hosting: Self-hosting involves setting up your own web server and deploying the application to it. This is a more complex option, but it offers greater control over the deployment environment.
B. Testing the application to ensure it works as expected:
After the application has been deployed, the next step is to test it to ensure that it works as expected. Testing involves verifying that the application functions correctly and meets the requirements defined in the design phase.
-
Unit Testing: Unit testing involves testing individual components of the application to ensure that they function as expected. This can be done using a testing framework, such as Jest or Mocha.
-
Integration Testing: Integration testing involves testing the application as a whole to ensure that all components work together as expected. This can involve testing the integration between the ReactJS application and the Zillow API.
-
User Acceptance Testing: User acceptance testing involves testing the application from the user's perspective to ensure that it meets their needs and requirements. This can involve getting feedback from users and making changes to the application as necessary.
C. Debugging and fixing any issues:
Debugging is the process of identifying and fixing issues with the application. During the testing phase, any issues that are discovered should be fixed in a timely manner to ensure that the application functions correctly.
-
Debugging Tools: There are several debugging tools available for ReactJS applications, including the React Developer Tools, which are built into the Google Chrome browser. These tools allow developers to inspect the state of the application and identify any issues.
-
Fixing Issues: Once an issue has been identified, the next step is to fix it. This can involve making changes to the code, updating dependencies, or modifying the configuration of the application.
In conclusion, deployment and testing is an important step in the development of a ReactJS application. It involves deploying the application to a web server, testing it to ensure it works as expected, and debugging and fixing any issues that are discovered.
Conclusion:
In this framework, we have covered the process of building a real-estate listing application using ReactJS and the Zillow API. We have discussed the benefits of using the Zillow API, setting up the ReactJS environment, building the ReactJS application, and deploying and testing the application. By following this framework, you can build a robust and user-friendly real-estate listing application that leverages the power of ReactJS and the Zillow API.
If you need help with the development of a real-estate listing application, hire react js developers. ReactJS developers have the expertise and experience needed to build high-quality applications that meet the needs of your users. With their help, you can ensure that your application is developed quickly and efficiently, and that it functions as expected. Whether you need help with the design, development, or deployment of your application, hiring ReactJS developers can be a valuable investment that will help you achieve your goals and achieve success.